We will demonstrate how to use a decision tree model in Python to predict exoplanetary orbit periods based on features such as exoplanetary mass, radius, and orbit distance.
We will start by importing the necessary libraries, including pandas
for reading the data from a CSV file, matplotlib
for visualizing the results, and DecisionTreeRegressor
and train_test_split
from scikit-learn for building and evaluating the model.
Next, we will load the data from a CSV file using pandas
and select the relevant columns. We will then split the data into training and testing sets, with 80% of the data being used for training and 20% being used for testing.
Next, we will train the decision tree model on the training data using the fit
method. This method “fits” the model to the data, which means it learns patterns in the data that can be used to make predictions.
We will then test the model on the testing data using the predict
method, which generates a set of predictions for the exoplanetary orbit periods. These predictions will be compared to the actual exoplanetary orbit periods to evaluate the model’s performance.
Finally, we will visualize the model’s performance using a scatter plot, which shows the relationship between the actual exoplanetary orbit periods and the predicted orbit periods. Points that are close to the diagonal line indicate more accurate predictions.
Overall, this demonstration shows how a decision tree model can be used to make predictions about exoplanetary orbit periods based on other features of the exoplanets. This type of analysis could be useful for understanding the properties of exoplanets and how they may vary within our galaxy and beyond.
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Mon Jan 2 05:24:38 2023
@author: ramnot
"""
# Import necessary libraries
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.tree import DecisionTreeRegressor
from sklearn.model_selection import train_test_split
# Load the data from the CSV file
df = pd.read_csv("data.csv")
# Select the relevant columns
X = df[["exoplanetary mass", "exoplanetary radius", "exoplanetary orbit distance"]]
y = df["exoplanetary orbit period"]
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the decision tree model
model = DecisionTreeRegressor()
model.fit(X_train, y_train)
# Test the model on the testing data
predictions = model.predict(X_test)
# Evaluate the model's performance
from sklearn.metrics import mean_absolute_error
mae = mean_absolute_error(y_test, predictions)
print(f"Mean Absolute Error: {mae:.2f}")
# Plot the predictions and actual values
plt.scatter(y_test, predictions)
plt.xlabel("Actual Values")
plt.ylabel("Predictions")
plt.title("Predictions vs Actual Values")
plt.show()
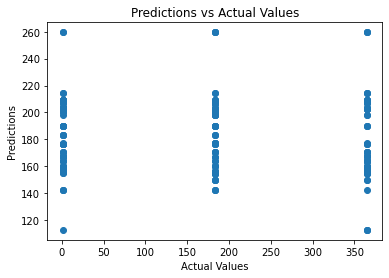
RAMNOT’s Build:
- Identifying and characterizing potentially habitable exoplanets that could support life.
- Studying the atmospheres of exoplanets to better understand their chemical compositions and potential for supporting life.
- Using exoplanet data to understand the formation and evolution of planetary systems in the universe.
- Searching for exoplanets that could be used as waypoints for interstellar travel.
- Analyzing exoplanetary orbits to learn more about the gravitational forces at play in planetary systems.
- Studying the demographics of exoplanetary systems to learn about the prevalence of different types of planets.
- Using exoplanet data to test and refine theories about the formation and evolution of planetary systems.
- Searching for exoplanets that could be used as resources for space exploration and colonization.
- Analyzing exoplanetary atmospheres to learn about the conditions and processes that give rise to them.
- Using exoplanetary data to learn about the prevalence and properties of “rogue” planets that do not orbit any star.
- Studying the composition and structure of exoplanetary surfaces to understand the geology and geochemistry of other worlds.
- Searching for exoplanets that could be used as laboratories for studying fundamental physics.
- Analyzing exoplanetary atmospheres to learn about the climate and weather patterns on other worlds.
- Using exoplanetary data to learn about the prevalence of Earth-like planets in the universe.
- Studying the exoplanetary data to learn about the prevalence and properties of “super-Earths,” which are exoplanets with masses between 1 and 10 times that of Earth.
- Analyzing exoplanetary data to learn about the prevalence and properties of “mini-Neptunes,” which are exoplanets with masses between 10 and 100 times that of Earth.
- Searching for exoplanets that could be used as laboratories for studying the origins and evolution of life.
- Analyzing exoplanetary data to learn about the prevalence and properties of “gas giants,” which are exoplanets with masses hundreds of times that of Earth.
- Using exoplanetary data to learn about the prevalence and properties of “exoplanetary moons,” which are moons orbiting exoplanets.
- Analyzing exoplanetary data to learn about the prevalence and properties of “exomoons,” which are moons orbiting exoplanets.