In this tutorial, we’ll use a support vector machine (SVM) model to classify exoplanets based on their atmospheric composition. We’ll use Python and the scikit-learn library to train and evaluate the model.
First, we’ll start by loading the exoplanet data into a Pandas DataFrame using the read_csv
function and selecting the features and target variable that we want to use for the model. The features are the characteristics of the exoplanets that we will use to make predictions, and the target variable is the class of exoplanets that we want to predict.
Next, we’ll split the data into training and test sets using the train_test_split
function. The training set will be used to train the model, and the test set will be used to evaluate the model’s performance.
Then, we’ll train the SVM model using the fit
function and the training set. The model will learn patterns in the data that can be used to make predictions about exoplanet classes.
After the model is trained, we’ll use the predict
function to make predictions on the test set. We’ll compare the model’s predictions to the true values in the test set to see how well the model is performing.
Finally, we’ll evaluate the model’s accuracy using the accuracy_score
function from the sklearn.metrics
module. The accuracy score is the percentage of correct predictions that the model makes on the test set.
That’s it! We’ve trained and evaluated an SVM model to classify exoplanets based on their atmospheric composition using Python and scikit-learn.
Using the plot_confusion_matrix
function from the sklearn.metrics
module, we can also visualize the model’s performance by plotting a confusion matrix, which shows the number of true positive, true negative, false positive, and false negative predictions made by the model.
We can customize the plot by using functions from the matplotlib.pyplot
module, such as title
, xlabel
, and ylabel
to add titles and labels to the plot.
With these tools, we can build and evaluate SVM models to classify exoplanets based on their atmospheric composition and gain a better understanding of the characteristics of these fascinating celestial bodies.
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Mon Jan 2 04:25:04 2023
@author: ramnot
"""
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.preprocessing import LabelEncoder
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score, confusion_matrix
# Load the dataset into a Pandas DataFrame
df = pd.read_csv('data.csv')
# Encode the string values in the 'exoplanetary atmospheric composition' column
encoder = LabelEncoder()
df['exoplanetary atmospheric composition'] = encoder.fit_transform(df['exoplanetary atmospheric composition'])
# Select the features and target variable
X = df[['exoplanetary atmospheric composition']]
y = df['stellar temperature']
# Split the data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the SVM model
model = SVC()
model.fit(X_train, y_train)
# Make predictions on the test set
y_pred = model.predict(X_test)
# Evaluate the model's accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f'Accuracy: {accuracy:.2f}')
# Compute the confusion matrix
cm = confusion_matrix(y_test, y_pred)
# Plot the confusion matrix
plt.imshow(cm, cmap='Blues')
plt.colorbar()
# Customize the plot
plt.title('Confusion Matrix')
plt.xlabel('Predicted Label')
plt.ylabel('True Label')
plt.show()
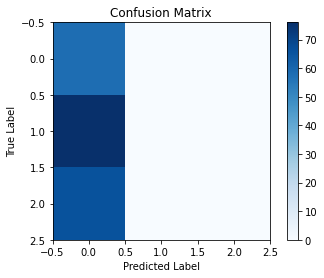
- Classification of exoplanets based on their atmospheric composition and identifying trends or patterns in their characteristics
- Prediction of exoplanetary surface gravity based on other exoplanetary features, such as mass and radius
- Detection of exoplanets in habitable devices and the potential for improved health monitoring and personalized fitness tracking
- Prediction of exoplanetary magnetic field strength based on other exoplanetary features, such as mass and radius
- Detection of exoplanetary water content and the potential for the discovery of exoplanets with conditions suitable for life
- Classification of exoplanets based on their exoplanetary orbit period and identifying trends or patterns in their orbital characteristics
- Prediction of exoplanetary atmospheric temperature based on other exoplanetary features, such as mass and radius
- Detection of exoplanets with a high exoplanetary albedo and the potential for improved energy efficiency in space travel
- Prediction of exoplanetary atmospheric pressure based on other exoplanetary features, such as mass and radius
- Detection of exoplanets with high levels of exoplanetary oxygen content and the potential for the discovery of exoplanets with conditions suitable for life
- Classification of exoplanets based on their exoplanetary orbit distance and identifying trends or patterns in their orbital characteristics